Top Exciting Spring Boot Projects & Topics For Beginners in 2021
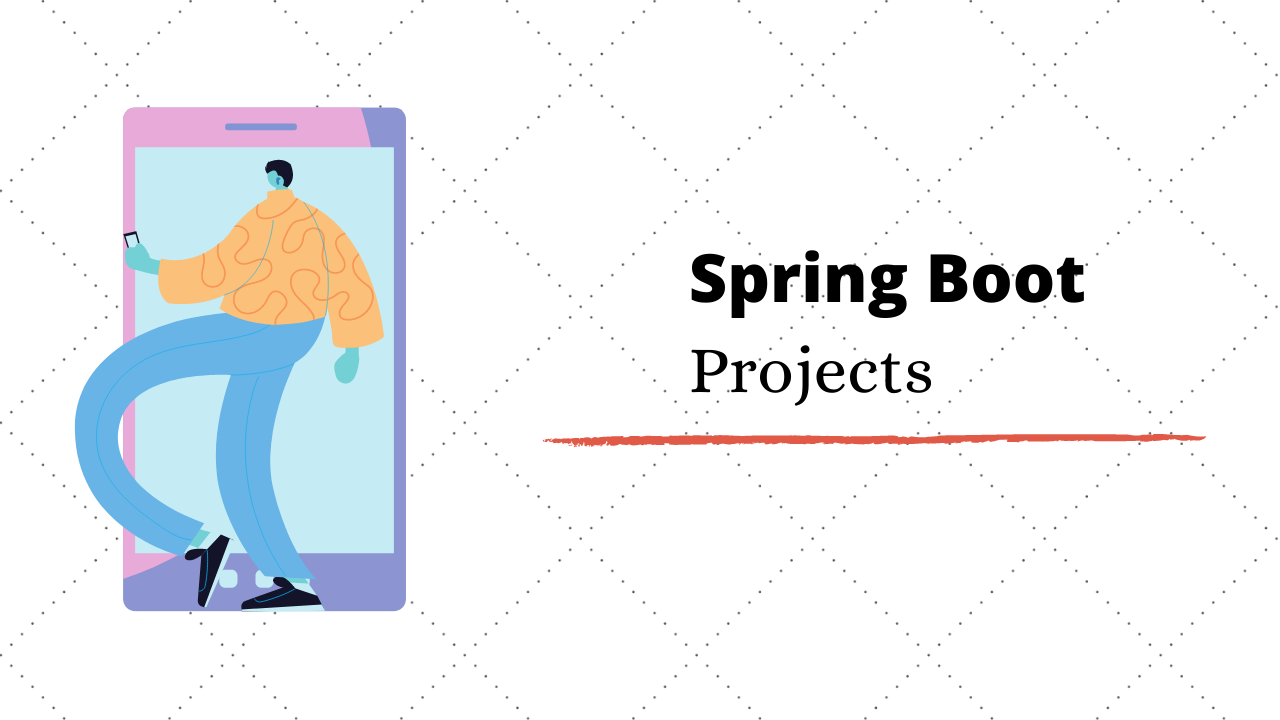
One of the best ways to be taught any technology is by utilizing it. So, if you wish to strengthen your information of Spring Boot, you need to work on a couple of Spring Boot projects. A major challenge with projects is it’s fairly difficult to give you their ideas.
To help you there, we’ve compiled an inventory of Spring Boot project ideas. You possibly can choose any one of the ideas we’ve shared right here so you’ll be able to take a look at your knowledge.
However we earlier than discussing the projects, let’s take a look at the fundamentals of Spring Boot:
What’s the Spring Boot?
Spring Boot is an open-source framework primarily based on Java, which permits users to create micro Services. A microservice permits developers to develop and ship services independently. Right here, each service has its course of, which reinforces the light-weight model. Spring means that you can develop a production-grade stand-alone application you’ll be able to run. You can begin your process without performing the disturbing configuration setup of Spring.
That’s why it’s broadly widespread amongst Java developers. Creating and understanding spring purposes is comparatively straightforward. Furthermore, because it removes the complicated configuration process, you develop and deploy applications sooner with greater effectivity and productiveness.
Let’s now concentrate on different Spring Boot project ideas:
Spring Boot Project Ideas
1. Use Spring Boot to construct a Web Application
This can be a beginner-level project. You can begin your Spring learning journey by making a easy web application. You’ll use both Maven 3.2+ or Gradle 4+. You’ll first need to create a application class then run the applying. You’ll even have so as to add unit assessments. For starters, you’ll be able to construct a website for a enterprise. Aside from application class and unit assessments, your web application would require services too. So as to add services, you’ll have to make use of the actuator module of Spring Boot.
Right here’s the pattern code for creating the application class:
package com.example.springboot;
import java.util.Arrays;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class Application {
public static void main(String[] args)
SpringApplication.run(Application.class, args);
@Bean
public CommandLineRunner commandLineRunner(ApplicationContext ctx) {
return args -> {
System.out.println(“Let’s examine the beans supplied by Spring Boot:”);
String[] beanNames = ctx.getBeanDefinitionNames();
Arrays.sort(beanNames);
for (String beanName : beanNames) {
System.out.println(beanName);
}
};
}
}
To search out out extra about this project, you’ll be able to go here.
After you’ve created a web application with Spring Boot, you’ll be aware of the fundamentals of this highly effective tool. You possibly can take this a step additional by including extra options to your web app and improve its performance.
2. Create REST Service for an Education Site
On this challenge, you’ll create a REST service for a course-providing web site. REST is an abbreviation for Representational State Transfer, and to create these services; you’ll use Spring Boot. For instance, one service can ask for the courses a specific scholar signed up for, through the Get request methodology. Different service examples embrace asking for the scholars of a particular class, in addition to a request to register a scholar.
To finish this project, you’ll want Maven 3.0+, an IDE, and JDK 1.8+. You also needs to be aware of the Richardson Maturity Model as you’ll want that to establish the extent of maturity of your Restful Web Service. This project will enable you to get extra aware of REST and its implementation via Spring Boot.
REST offers architectural constraints to services, that are known as RESTful providers. These constraints comprise of Cacheable outcomes (corresponding to HTTP cache), uniform URL, presence of a service shopper and producer, and statelessness.
You need to use the Spring Web MVC as your web framework. To bootstrap this project (in addition to different Spring Boot projects), you should use Spring Initializr. You’d have to decide on the Group, the Artifact, and Web, Actuator, and DevTools as your dependencies there. Your service would require information too. For that function, you should use an in-memory retailer corresponding to “ArrayList”.
You’ll additionally get accustomed to utilizing varied request strategies whereas finishing this undertaking since you’ll have to make use of GET, PUT, POST, and DELETE.
3. Create an Employee Administration System (Utilizing Spring Boot and Thymeleaf)
Through the use of Spring Boot and Thymeleaf, you’ll be able to construct an employee administration system for a company. However for this, you have to be aware of the fundamental ideas of each of those technologies. The consumer of your EMS solution ought to have the ability to add an employee, view all staff, delete any employee, kind the information, and paginate.
It’s a fairly complicated project which may take you a while to complete. Nonetheless, once you’re done, you’d be aware of the Thymeleaf CRUD, Spring Boot, and their varied functionalities in dealing with databases.
You’ll have to make use of an IDE, Spring Data JPA, Spring Boot 2.2, Maven 3.2+, and Spring Framework 5.2. It is best to divide it into steps and full them one-by-one. Begin with organising the database, after which start including the required options.
4. Work on the Open-source Project Sagan
Project Sagan is a real-world app that facilitates the official website of Spring Boot (spring.io). It’s obtainable on Github and is a well-liked open-source undertaking. By engaged on this undertaking, you’ll get to be taught rather a lot in regards to the capabilities of Spring Boot whereas additionally getting aware of its framework, Elasticsearch, Thymeleaf UI, and Gradle.
Because it powers an actual website, you’ll be able to see its outcomes anytime. You possibly can see the progress of different developers and the way they’ve worked on this project as effectively.
It’s an effective way to expertise the assorted functionalities of Spring Boot.
5. Create a Web App with Spring Boot Starter Web
Spring Boot starters can assist you do away with many complicated steps current within the development process. They’re dependency descriptors you’ll be able to add in your software and get all the advantages of Spring. Spring Boot Starter Web is a well-liked starter. It means that you can construct an app with restful services.
On this project, you’ll first have to make use of the Spring Initializr to bootstrap the project. Then, you’ll add Spring Boot Starter Web. It performs auto-configuration and provides the mandatory dependencies you’d want for any web application.
This implies your undertaking gets dependencies for Tomcat, Validation API, beans, MVC, and lots of others. As you’ll be able to see, by utilizing Spring Boot Starter Web, you’ll be able to simply make the event course of extra productive.
This was simply an instance, and you should use many different Spring Boot starters and improve your outcomes.
Conclusion
Spring Boot is definitely probably the most widespread tools amongst Java developers and back-end developers.